After the previous post about the mandelbrot renderer written in Go, this article will be related to the same topic, only this time i'll talk about a terminal based Julia set generator written again in Go language. In fact i've done this experiment prior to write the mandelbrot renderer. About the implementation I've discussed in the last article.
The whole idea came from the motivation to create something which resembles the feeling which only a terminal based application can create (something which is close enough to the pixelart technique), but at the same time it has enough visually appealing characteristics and above all it's dynamic.
Into the terminal fractals
(In preview the ASCII Mandelbrot generator running in terminal)
Because in the last few months i was pretty much delved into the fractals, i've started to create some small experiments, one of them being the code snippet below:
https://gist.github.com/esimov/970a3816dda12e4059e3.js
Which produce the following terminal output:
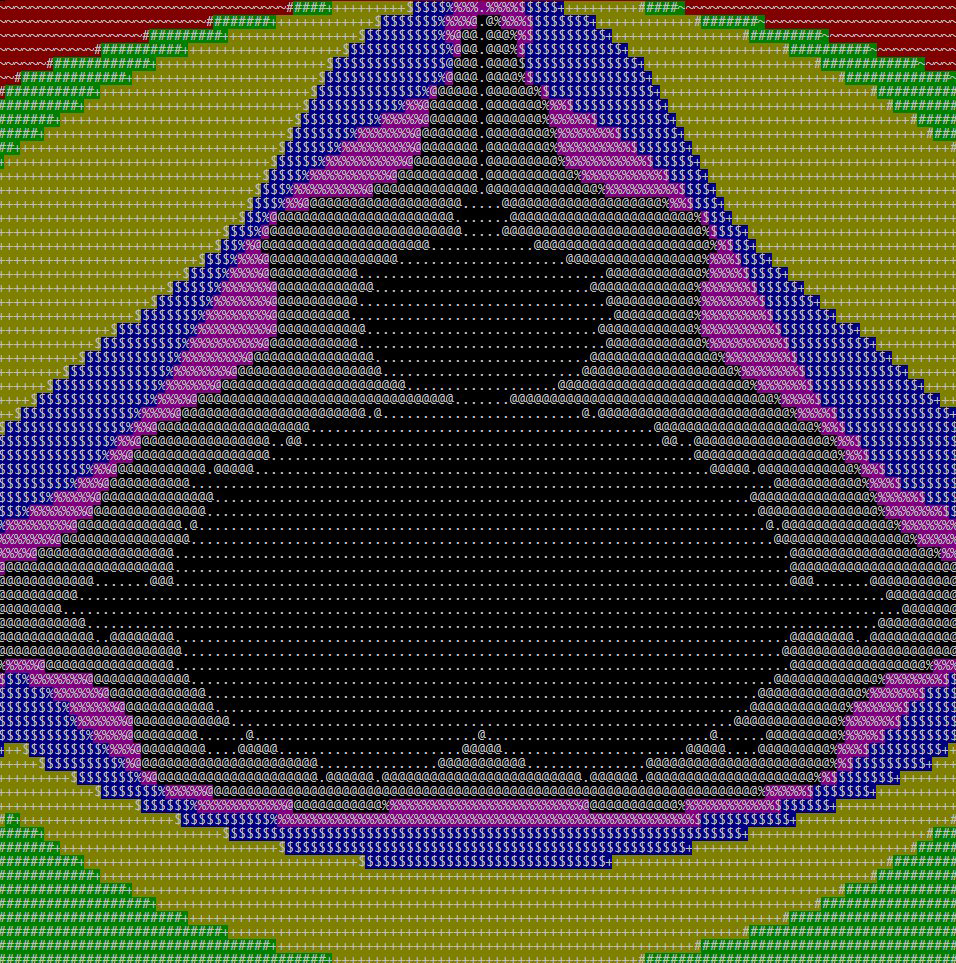
This was nice but i wanted to be dynamic, and not something simply put in the terminal window. So i've started to search for methods to refresh the terminal window periodically, ideally to refresh on each mandelbrot iteration. For this reason i've created some utility methods to get the terminal window width and height. And another method to flush the screen buffer periodically.
// Get console width
func Width() int {
ws, err := getWinsize()
if err != nil {
return -1
}
return int(ws.Row)
}
// Get console height
func Height() int {
ws, err := getWinsize()
if err != nil {
return -1
}
return int(ws.Col)
}
// Flush buffer and ensure that it will not overflow screen
func Flush() {
for idx, str := range strings.Split(Screen.String(), "\n") {
if idx > Height() {
return
}
output.WriteString(str + "\n")
}
output.Flush()
Screen.Reset()
}
It was missing another ingredient: in terminal based application we need somehow to specify the cursor position where to output the desired character, some kind of pointer to a position specified by width and height coordinate. For this i've created another method which move the cursor to the desired place, defined by x and y:
// Move cursor to given position
func MoveCursor(x int, y int) {
fmt.Fprintf(Screen, "\033[%d;%dH", x, y)
}
Then we can clear the screen buffer periodically. In linux based systems to move the cursor to a specific place in terminal window we can use ANSI escape codes, like: \033[%d;%dH, where %d;%d we can replace with values obtained from the mandelbrot renderer. In the example provided in the project github repo i'm moving the terminal window cursor to the mandelbrot x and y position, after which i'm clearing the screen.
To make it more attractive i used a cheap trick to zoom in and out into the fractal and to smoothly displace the fractal position by applying sine and cosine function on x and y coordinate.
for {
n += 0.045
zoom += 0.04 * math.Sin(n)
asciibrot.DrawFractal(zoom, math.Cos(n), math.Sin(n)/zoom*0.02, math.Sin(n), MAX_IT, true, isColor) //where math.Cos(n) and math.Sin(n) are x and y coordinates
}
But there is another issue. We need to handle differently the code responsible to obtain the terminal window size in different operating systems. In linux and darwin based operating systems here is how we can get the terminal size:
func getWinsize() (*winsize, error) {
ws := new(winsize)
var _TIOCGWINSZ int64
switch runtime.GOOS {
case "linux":
_TIOCGWINSZ = 0x5413
case "darwin":
_TIOCGWINSZ = 1074295912
}
r1, _, errno := syscall.Syscall(syscall.SYS_IOCTL,
uintptr(syscall.Stdin),
uintptr(_TIOCGWINSZ),
uintptr(unsafe.Pointer(ws)),
)
if int(r1) == -1 {
fmt.Println("Error:", os.NewSyscallError("GetWinsize", errno))
return nil, os.NewSyscallError("GetWinsize", errno)
}
return ws, nil
}
In Windows operating system to obtain the terminal dimension is a little bit different:
type (
coord struct {
x int16
y int16
}
consoleScreenBufferInfo struct {
size coord
cursorPosition coord
maximumWindowSize coord
}
)
// ...
func getWinSize() (width, height int, err error) {
var info consoleScreenBufferInfo
r0, _, e1 := syscall.Syscall(procGetConsoleScreenBufferInfo.Addr(), 2, uintptr(out), uintptr(unsafe.Pointer(&info)), 0)
if int(r0) == 0 {
if e1 != 0 {
err = error(e1)
} else {
err = syscall.EINVAL
}
}
return int(info.size.x), int(info.size.y), nil
}
One last step remained: to clear the screen buffer on CTRL-C signal, in another words to clear the screen on control break. For this i've created a channel and when the CTRL-C was pressed i signaled this event and on a separate goroutine i was listening for this event, meaning i could break the operation.
// On CTRL+C restore default terminal foreground and background color
go func() {
<-c
fmt.Fprint(asciibrot.Screen, "%s%s", "\x1b[49m", "\x1b[39m")
fmt.Fprint(asciibrot.Screen, "\033[2J")
asciibrot.Flush()
os.Exit(1)
}()
Usage
In big that's all. You can grab the code by running the following command:
go get github.com/esimov/asciibrot
To run it type:
go run julia.go --help
Terminal fractal ASCII art written in Go. #golang #fractal #terminal #ascii pic.twitter.com/WtwHkhoOPz
— Simó Endre (@simo_endre) March 28, 2016
You can run the example in monochrome or color version. For the color version use --color or -c. For monochrome version use --mono or -m.
You can build the binary version with: go build github.com/esimov/asciibrot.
I've created a github repo for this experiment, which you can get it here: https://github.com/esimov/asciibrot